First, don't feel bad, this lesson's instructions are kind of confusing. You can skip to what you need if you want.
4 Posts:
1.) Explaining For Loops
2.) Exercise 5/7's if statement
3.) Exercise 5/7's inner loop
4.) Exercise 5/7's push statement
1.) Explaining For Loops
2.) Exercise 5/7's if statement
3.) Exercise 5/7's inner loop
4.) Exercise 5/7's push statement
1.) First you need to understand how for loops work.
For loops are really useful for doing something over and over. Example: For every horse in the barn, tell me the horse's name and it's age. You'll be going to a horse over and over again to get it's name and age. But computers are stupid so you have to be reallyyyy specific about it, like this:
For ( every horse in the barn starting at the one in the first stall; until you get to the 12th stall; go to the next stall) { This is what I want you to do at every stall. Get it's name and age.}
Now for the computer to understand us we have to speak Javascript to it because it doesn't know English. In Javascript, that's a for loop:
Now for the computer to understand us we have to speak Javascript to it because it doesn't know English. In Javascript, that's a for loop:
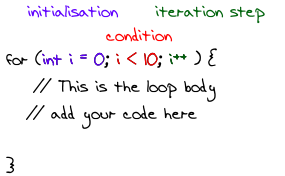
This is a bit confusing because the order this runs in is like this:
1.) Initialization: Tell the computer where to start (here it says start with i = 0. So at zero.)
2.) Condition: This is kind of like an if statement...you have to check "If this condition is true...then do the loop body." ( while i is less than 10)
3.) Loop Body: you can make this do whatever you want, like get the horse's name
4.) Iteration step: After you do the loop body, do this. Then go back to the condition and see if it's still true (i++ means adding 1 to i. Remember, i = 0 from the initialization. So it becomes i = 1. You start the for loop over at the condition, and i is still less than 10, so you do the loop body again....basically you just keep doing condition, loop body, iteration over and over until the condition is no longer true. That's why it's called a loop.)
So let's look at the loop from the "Search Text For Your Name" exercise:
for(var i = 0; i < text.length; i++) {
// Do stuff
}
Closer to English, that's this:
For (every i, starting at i = 0 ; while i is less than the length of the text; after the loop
body, add 1 to i.) {
// This is the loop body
}
So far, the code will keep increasing i by 1 until it's no longer less than the length of the text. So for example, this code:
text = "Example"
for(var i = 0; i < text.length; i++) {
console.log(i)
}
That will print out
0
1
2
3
4
5
6
0
1
2
3
4
5
6
the word "example" is 7 letters long but remember it starts counting at 0.
Look at the code and see if that part makes sense to you. If it still doesn't, try Googling "Javascript for loops" and look over explanations/examples until you feel comfortable with for loops. Practice makes perfect, and this is a core concept you really need to be comfortable with.
2.)
for(var i = 0; i < text.length; i++) {
if (text[i] === "J") {
// Do stuff
}
}
for(var myNumber = 0; myNumber < text.length; myNumber++) {
if (text[myNumber] === "J") {
// Do stuff
}
}
text =" My name is Jem."
for(var myNumber = 0; myNumber < text.length; myNumber++) {
console.log(myNumber)
if (text[myNumber] === "J") {
console.log("I found a J!")
}
}
3.)
for(var i = 0; i < text.length; i++) {
if (text[i] === "J") {
for(var j = i; j < (myName.length + i); j++) {
hits.push(text[j]);
}
}
}
for(var k = i; k < (myName.length + i); k++) {
// This is the loop body
hits.push(text[k]); }
For(start at k. We'll make k equal to i, which is whatever we're at in the first for loop;
Keep looping while k is less than the length of (the length of my name, plus the number we're at with the first for loop.)
after we do the loop body, we'll increase k by 1)
4.)
// This is the loop body
hits.push(text[k]); }
var text = "Hey, how are you \
doing? My name is Jem. Did you know that I am called Jem? Yeah. Cause it's \
true. I'm Jem. Woohoo. Text and stuff.";
var myName = "Jem";
var hits = [];
for(var i = 0; i < text.length; i++)
{
if (text[i] === "J")
{
for(var j = i; j < (myName.length + i); j++)
{
hits.push(text[j]);
}
}
}
Thanks a lot for plodding through this. It was very helpful to have it broken down into digestible parts.
ReplyDeleteI'm glad you found it helpful! :)
DeleteVery nice, Jemmeh!! Congrats :))
ReplyDeleteThank you, Tony!
DeleteThank you so much! The horse analogy really helped me understand for loops. I've copied it down into my notebook.
ReplyDeleteThanks a lot, Jem! I hope you can also expand on those other sections that were left over.
ReplyDeleteThis was an essential read. Looking forward to any more to come. Thanks!
ReplyDeleteThanks Jemmeh, it was super helpful!
ReplyDeleteFor the record when you write this:
ReplyDeletefor(var j = i; j < (myName.length + i); j++)
Make sure you're using the right letter. For example, in Jem "j" is okey, but for Yan you need "y":
for(var y = i; y < (myName.length + i); y++)
Thank you so much this helped a lot!!
ReplyDeleteSo glad you found it useful! :)
ReplyDelete